Jboss tools has a rich toolset for Rapid Application Development using Eclipse. I suggest you download the Jboss Tools (3.0 as on date of this post) and install it in eclipse. The installation instructions are given online. I downloaded the Zip file and copied it over to my plugins folder for Eclipse. Here is the link for Jbosss Tools
http://www.jboss.org/tools/download
Dependencies
You need to download the RichFaces Library.
http://www.jboss.org/jbossrichfaces/downloads/
Jboss Tools
http://www.jboss.org/tools/download
Eclipse 3.4 (Ganymede) for JEE Developers
http://www.eclipse.org/
Apache Tomcat
http://tomcat.apache.org/
JDK 1.6
Configuration
Since the Jboss tools is installed you would be able to easily create a new JSF Project using File > New Project > JSF Project .
This will start a wizard
Once the project is created Jboss Tools will automatically add the following libraries for you, just like shown below
Next go ahead and add the RichFaces Library from the ${RichFaces Unzipped Folder}\lib to the lib directory of the eclipse web application
You would also need the JSF 1.2 library jars. You can download the jar files from the following location
https://javaserverfaces.dev.java.net/servlets/ProjectDocumentList?folderID=10411
We need the following 3 jar files from the JSF 1.2 Mojarra project
Add the following to the web.xml for the RichFaces Filters and Servlet prameters
Now the configuration part is over. Let's do some coding for the jsp page
Coding
The first thing we will do is to make sure that when the user hits the URL he gets redirected to our JSF page. In order to do this create a new JSP page bu right clicking webcontent folder File >JSP File . Choose the template as a JSPRedirect
Add the following lines to the jsp source
Next lets create new JSP page and set its Template as JSPBasePage using the above option. Switch the Workspace perspective to Web and as soon as the page is open you will see on the right hand side the RichFaces toolbox as shown below.
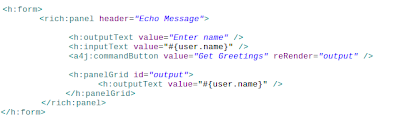
Next Add the Bean class User.java
We need to add the Bean to the Managed Beans section of faces-config.xml file
Once this is done we are ready to run the project. Go ahead and right click on the project in the Package Exlporer and choose Run As > Run On Server. This will bring the echo.jsf file
Here is the output of the project. The echo.jsf page does a AJAX request and refreshes the value inside the PanelGrid component.
until next post :)